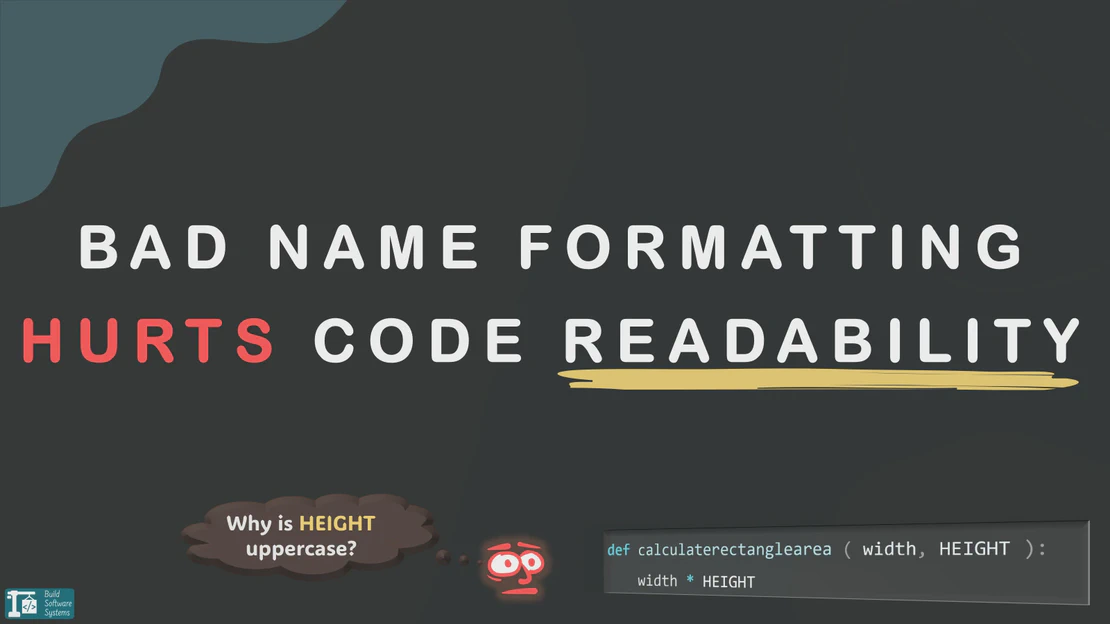
You Should Format Names in Your Code
- March 24, 2025
- 6 min read
- Programming concepts
Table of Contents
In my code, I need to define a variable to represent “my new item”. But how should I name it? Should I write it as: “mynewitem” or “myNewItem” or “MyNewItem” or “MYNEWITEM” or “MY_NEW_ITEM” or “My_New_Item” or “my_New_Item” or “my_new_item”?
Does it even matter how I format the variable name—or any other code item? Absolutely—yes!
Just like in the English language, the way you format code names significantly impacts readability.
In this article, I’ll discuss why formatting matters and how you should format code names properly.
​
The Importance of Naming Conventions
Consider these two functions from CPython, the python language interpreter:
PyList_SetItem
PyList_SET_ITEM
At first glance, they both seem to represent ‘Py List Set Item’. But in reality, they serve different purposes:
PyList_SetItem
is a function.PyList_SET_ITEM
is a macro.
How do we know that? CPython follows this convention consistently, making it easy to distinguish functions from macros at a glance.
Additionally:
PyList_SetItem
performs error checking.PyList_SET_ITEM
does not (which is a common pattern for CPython macros).
See how much you can infer just from the name—without even checking the documentation?
That’s the power of a good naming format.
Free Naming Style Cheat Sheets
Get a free cheat sheet with naming styles for popular programming languages and receive content updates
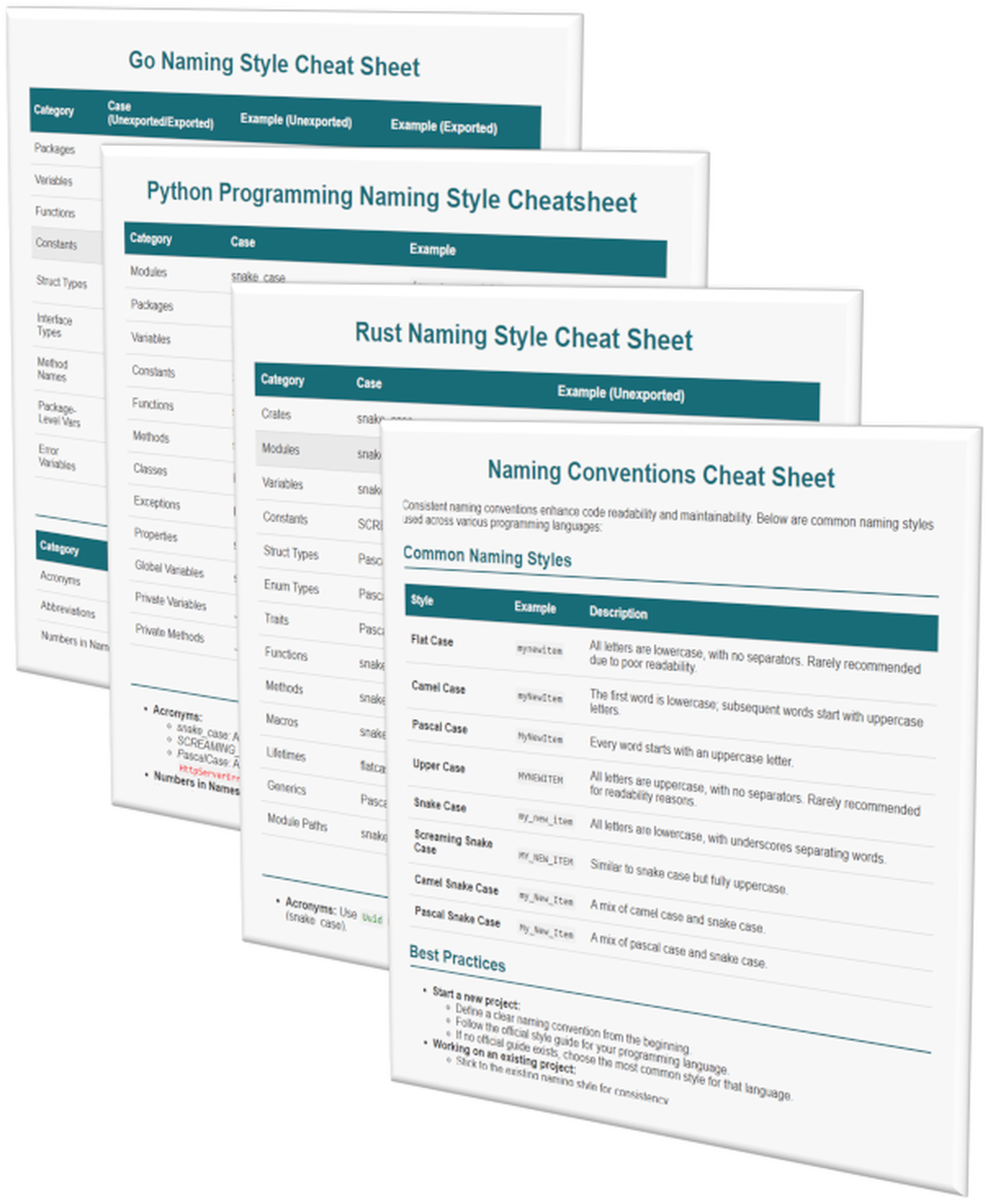
​
How Should You Format Your Names?
​
Step 1: Describe What the Item Represents
Before worrying about format, turn the item’s purpose into plain English.
For example:
- A function that fetches user data → “fetch user data”
- A constant for maximum retries → “max retries”
You can find more detailed guidelines in my Descriptive Variable Names Are Not Always Good article and the following articles 1 2 3 4 5.
​
Step 2: Apply the Correct Format
Now, take your description and convert it into a proper code-friendly format.
Here are common naming styles and when to use them:
-
Flat Case (
calculatetotal
)- All lowercase, no separators.
- Rarely recommended due to poor readability.
-
Camel Case (
parseHtmlInput
)- First word is lowercase, others start with uppercase.
- Common in JavaScript functions.
-
Pascal Case (
DatabaseConnection
)- Every word starts with an uppercase letter.
- Used for class names in Python, Java, and C#.
-
Upper Case (
MAXVALUE
)- All uppercase, no separators.
- Rarely recommended for readability reasons.
-
Snake Case (
max_retries
)- Words are lowercase, separated by underscores.
- Common in Python variables and C macros.
-
Screaming Snake Case (
DEFAULT_TIMEOUT
)- Like snake case, but fully uppercase.
- Often used for constants in Python and Java.
-
Camel Snake Case (
http_Response_Code
)- A mix of camel case and snake case.
- Rare but used for logical grouping.
-
Pascal Snake Case (
Xml_Parser_Config
)- A mix of Pascal case and snake case.
- Sometimes used in APIs.
-
Kebab Case (
background-color
)- Words are lowercase, separated by hyphens.
- Common in CSS class names and URLs. It’s not valid in most programming languages.
-
Screaming Kebab Case (
API-ENDPOINT
)
- Like kebab case, but fully uppercase.
- Often used for environment variables. It’s not valid in most programming languages
- Train Case (
User-Profile-Page
)
- Words are capitalized, separated by hyphens.
- Common in file names, like
Project-Report-2023.pdf
. It’s not valid in most programming languages.
Note
There’s no single rule for handling numbers or acronyms in variable names.
For example:
- PascalCase:
XmlParserConfig
vs.XMLParserConfig
- Snake Case:
value1
vs.value_1
Each style guide should pick one and stick to it.
Some projects, like CPython, even combine formats to create a unique naming style.
For instance, PyList_CheckExact
is a blend of PascalCase and snake_case—helping group related functions together in C, which lacks built-in namespaces.
​
Language-Specific Naming Rules
Some programming languages, like Go, enforce naming conventions at the language level. In Go, capitalization alone determines whether a variable is public or private.
example.go (module being used)
1package example
2
3// Exported (public) because it starts with an uppercase letter
4var MyItem = "I am visible outside the package"
5
6// Not exported (private) because it starts with a lowercase letter
7var myItem = "I am not visible outside the package"
main.go (importing the module)
1package main
2
3import (
4 "fmt"
5 "example"
6)
7
8func main() {
9 // ✅ Works fine
10 fmt.Println(example.MyItem)
11
12 // ❌ Will cause a compile-time error
13 // fmt.Println(example.myItem)
14}
in example.go, MyItem
is exported, while myItem
is not.
This simple rule makes it crystal clear which items belong to the public API.
​
Best Practices for Naming Conventions
​
Starting a New Project?
- Define a clear naming convention from the beginning.
- Follow the official style guide for your programming language.
- If no official guide exists, choose the most common style for that language.
​
Working on an Existing Project?
- Stick to the existing naming style for consistency.
- Don’t mix different naming formats unless necessary.
​
Need Help Choosing a Name?
- AI tools like github Copilot, Cursor, tabnine, CodeGeeX or Codeium can suggest names that match your style.
​
Final Thoughts
Naming conventions aren’t just a technicality—they are a foundation of clean, maintainable code.
1️ Pick a naming style.
2️ Stick to it.
3️ Enforce it across your project.
📌 Here are the recommended naming style for C#, Go, Python and Rust!
📌 Want a handy reference? Get our free naming convention cheat sheet delivered directly to your email! Simply enter your details below.
Free Naming Style Cheat Sheets
Get a free cheat sheet with naming styles for popular programming languages and receive content updates
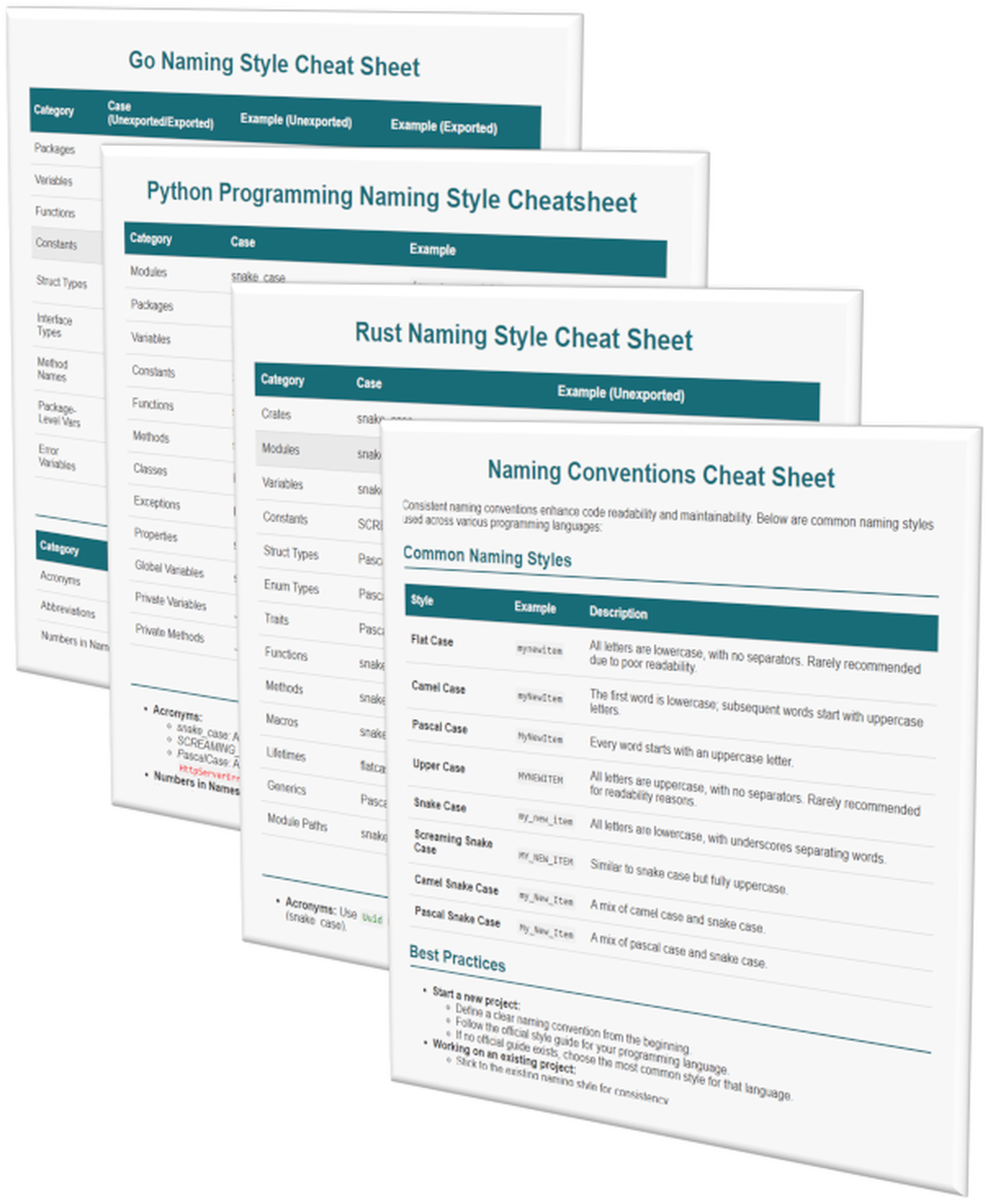