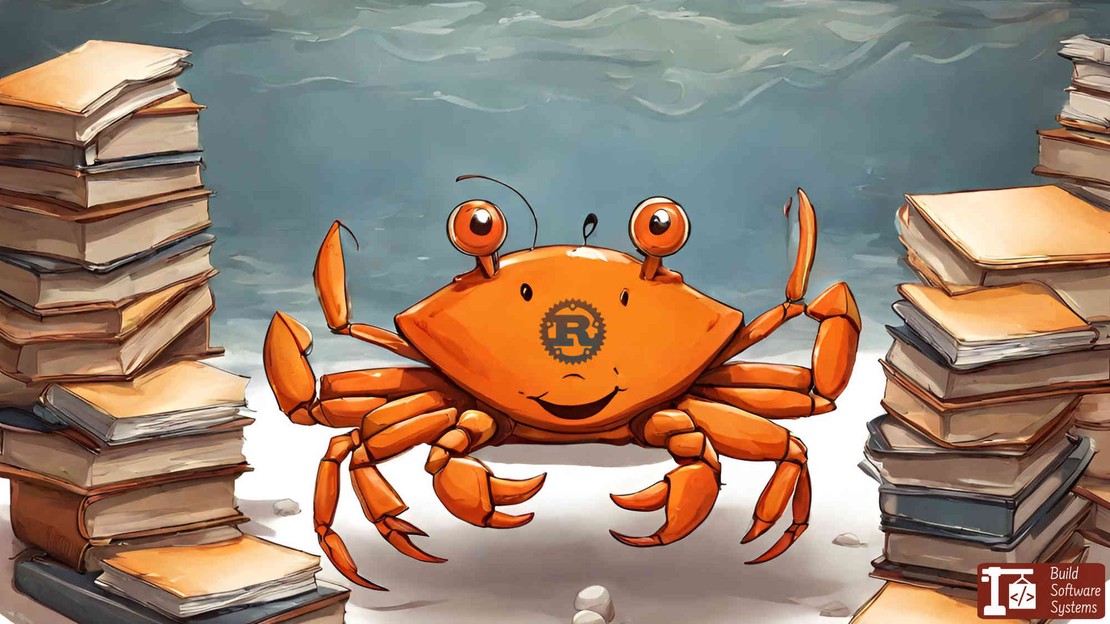
Rust Coding Conventions and Learning Resources
- April 22, 2024
- 10 min read
- Rust programming
Table of Contents
What coding style should I adopt for my Rust code to ensure consistency with my favorite Rust libraries? Where can I learn to develop a specific application using Rust and which libraries should I utilize? How can I make the most of Rust development tools?
Is there a specific place I can visit to find resources that will aid me in answering these questions?
This post serves as our index to resources that help developers like you master Rust and write excellent Rust code. If you haven’t started learning Rust yet, begin by:
- Reading the Rust programming language book.
- Keeping the Rust language cheat sheet handy for quick reference.
We’ll update the content as needed to include new relevant resources.
Newsletter
Subscribe to our newsletter and stay updated.
​
Idiomatic Coding Guidelines
Coding guidelines are essential because they improve code consistency, readability, and understanding. It’s easier to navigate an unfamiliar codebase when it resembles a familiar one.
Below are resources to help you make your Rust code idiomatic and adhere to Rust’s best practices.
​
API Design Guidelines
APIs (Application Programming Interfaces) are intended to be called by other programmers. Therefore, it’s crucial to design them for ease of use and maintenance. Below are relevant resources.
- Rust API Guidelines - Book: Recommendations on designing APIs for the development of idiomatic and interoperable Rust libraries.
- Elegant Library APIs in Rust (unofficial): A blog article listing tricks to improve Rust library code design.
- API Evolution Request for Comments: An official guide on handling API changes. It aids in determining and flagging API changes that could potentially affect users’ code.
​
Code Styling Guidelines
Code styling involves a set of formatting rules that define how code should appear. These rules cover indentation, function definition, line width, line breaks, and more. Consistent styling enhances code readability. The Rustfmt tool automatically formats Rust code according to a predefined idiomatic style. Below are resources detailing idiomatic Rust’s code style.
- Rust Style Guide: Established idiomatic Rust style guide.
​
Code Design Patterns
Design patterns dictate how to structure Rust code and leverage Rust’s characteristics to write productive, efficient, and easily understandable code. Explore the following resources to learn Rust design patterns:
- Rust Design Patterns (Unofficial): An mdBook offering good Rust code design practices as patterns. It demonstrates how to utilize various Rust features to ensure code is easily understandable and maintainable. The book covers Rust programming idioms, design patterns (recommended practices), and antipatterns (things to avoid).
- Effective Rust (Unofficial): An mdBook presenting many ways to enhance your Rust code. It provides insights on when to use macros and how to reason about lifetimes with generics. Additionally, it draws parallels with C++, which can be particularly beneficial for C++ developers.
- Rust Performance Pitfalls (Unofficial): A blog article highlighting both the best practices and common pitfalls for writing performant Rust code.
- The Rust Performance Book (Unofficial): An mdBook that provides “techniques that can improve the performance-related characteristics of Rust programs, such as runtime speed, memory usage, and binary size”.
​
Comments Guidelines
Comments enhance the clarity of code. Below are resources providing guidelines for commenting code in Rust:
- Rust Comments Convention - RFC 1574: Official guide to the idiomatic way to comment code.
- Comments Section of the Rust Book: Section of the Rust book that covers commenting in Rust.
- Comments Syntax Reference: Detailed reference on Rust comments syntax.
​
Documentation Guidelines
There are established methods for writing effective documentation for Rust programs. Below are recommendations for documenting Rust code idiomatically:
- Rust API Documentation Conventions Request for Comments - RFC 1574: Official API documentation guidelines recommendation. It replaces and builds upon the previous version RFC 505.
- Understanding Rust Documentation - the Rustdoc Book: Documentation of the Rustdoc tool, which generates Rust documentation.
​
Error Handling
Error handling is crucial in software development. Poor error handling can make code harder to read and introduce bugs. Here are resources that offer good practices for error handling in Rust code:
- Error Handling in Rust - Book (Unofficial): Advises on how to deal with errors in Rust code.
- Error Handling - from Old Rust Book: Walkthrough on dealing with errors in Rust, with numerous examples.
​
Naming Convention Recommendation
Consistency in naming program elements enhances code readability. The following guides provide Rust’s idiomatic approach to naming identifiers (variables, constants, function names, etc), types, and more.
- Rust naming Convention Request for Comments - RFC 430: A summarization of various naming conventions in Rust code. Further details about how these naming conventions were established can be found in RFC 344.
- Ownership Naming Conventions Request for Comments - RFC 199: Naming convention for variants of operation that access an object by value, by reference and by mutable reference.
- Rust API Naming: Naming convention that covers elements of the APIs not addressed in the naming convention specified in RFC 430. For instance, it includes guidelines for naming getters and setters.
​
Testing Guidelines
Do you test your code? Testing is essential in software development to quickly detect bugs introduced by changes and to facilitate code maintenance by other developers. Below are resources demonstrating how to organize tests for Rust programs and how to integrate testing into your workflow:
- General Testing Guide - Rust Book: The Testing section of the Rust book teaches how to organize and add tests to Rust projects.
- Testing Command Line Application (Unofficial): Guide with instructions on organizing and testing CLI applications, a part of the Rust CLI book.
Newsletter
Subscribe to our newsletter and stay updated.
​
Development and Tool Resources
Learning Rust to build various apps, knowing the right library crates for each problem, and mastering Rust’s development tools are all essential skills. These Rust learning resources can help you excel in all these aspects.
​
Resources by Application Type
Explore resources tailored to specific types of software applications.
​
Asynchronous Applications
Async programming facilitates the development of high-performance I/O-bound software.
- Asynchronous Programming in Rust (Async Book): Detailed guide on asynchronous programming in Rust and associated tools.
Rust has no built-in async runtime but relies on community-built runtimes like Tokio, Async-std and Smol.
- Tokio Async Runtime Tutorial: Official tutorial for getting started with Tokio.
- Tokio Async Runtime API Documentation: API documentation for Tokio.
- Tokio Async Internal Working: Official blog article explaining Tokio’s internal workings.
- Unit Testing Tokio Async-based Programs: Official guide for testing Tokio applications.
- Async-std Book: Official book on using the Async-std runtime.
- Smol Documentation: API documentation for the lightweight Smol runtime
​
Command Line Applications
Guides for developing CLI applications like terminal utilities.
- Command Line Applications in Rust - Book: Learn to build CLI applications in Rust.
- Test Command Line Application: Guide to organizing and testing CLI applications.
​
Embedded Software
Resources for using Rust in embedded systems development:
- The Embedded Rust Book: Official guide to Rust for embedded software.
- Aerospace and Robotics Resources: Categorized list of crates for aerospace engineering software.
- Clap Declarative Mode: User guide to use Clap in a declarative manner.
- Clap Imperative Mode: A user guide to use Clap imperatively.
​
Graphical User Interface (GUI)
Explore GUI frameworks.
- Choose Your GUI Framework: List of Rust GUI frameworks categorized by technologies and supported software platforms.
​
Graphics and Games
Resources for graphics and game development.
- Rust Game Development Ecosystem: Catalog of Rust crates for creating games.
- Game Development Resources Listing: Tutorials, videos, and books for learning game development with Rust.
- Sample Games Built Using Rust: Categorized list of games built with Rust, by the Rust community.
- Visual Effect (VFX) Ecosystem: Catalog of crates that specialize in various aspects of VFX production, from 3D rendering to animation, to quickly find the right one for your project.
- Visual Effect (VFX) Learning Resources: Compilation of videos, tutorials, articles and more to enhance your understanding of using Rust for VFX.
​
Machine Learning and Data
Rust community supports data analysis and machine learning.
- Rust Machine Learning Ecosystem: List of helpful Rust crates for different machine learning tasks like clustering, neural networks, and scientific computing.
​
Web Applications
In this connected world where remote services are increasingly prevalent, web applications continue to be in demand. Below are resources to learn how to develop web applications with Rust, along with popular frameworks to use.
- Rust Web Frameworks Overview Page: Information page about web frameworks and libraries in Rust.
- The Webassembly and Rust Book: A book about Rust and WebAssembly.
​
Tools and Libraries Learning
Explore resources that provide insights into various crates for different applications of Rust and help you better understand and utilize Rust development tools.
- The Unofficial Guide to the Rust Ecosystem lists popular crates for different Rust applications.
​
Build Systems, Package Management, and Other Tools
- Cargo Book: Official book for using Cargo, the Rust build and package management tool.
- Clippy Documentation: A guide covering the usage and development aspects of Clippy, the Rust linter.
- Clippy Lints Documentation: Detailed explanation of all the lint rules implemented in Clippy, categorized by Clippy version.
​
Development Environment
- Developing in an Offline Environment: Our article that guides you through the process of setting up (installing) the Rust toolchain and managing packages on a computer that is not connected to the Internet.
- Rustup Book: Official book for using Rustup, the Rust toolchain management tool.
- Visual Studio Code DevContainer Template for Rust: Template for Visual Studio Code development container to develop in Rust without installing the toolchain. Learn more about VSCode DevContainer templates here.
​
Rust Compiler
- Internal Closures Handling: Understand how the Rust compiler handles closures.
Newsletter
Subscribe to our newsletter and stay updated.
​
Extra
- The Rust Reference Book: The “primary reference for the Rust programming language”. You can find descriptions of Rust’s language features, how it functions, and the motivations behind its design.
- The Little Book of Rust Books: A curated list of official, unofficial and application-specific Rust books.
- Awesome Rust Books: A compilation of free and paid books for learning Rust programming and applying Rust for various purposes.
- List of Websites that Track the State of Rust in Related Areas: Links to websites tracking Rust’s progress in different domains.
- Awesome Rust: A comprehensive categorized list of Rust crates, along with learning resources such as books, podcasts, and blog articles.
- Rust-learning: A collection of links to various resources for learning Rust, including blogs, books, videos, podcasts, and more.
- Idiomatic-rust: Numerous resources for writing “ergonomic Rust code”.
- Rust CookBook: Tips on how to implement common programming tasks in Rust, covering various domains.
Do you use relevant Rust programming resources that haven’t been mentioned in this post?
Feel free to share them in the comments section or let us know.