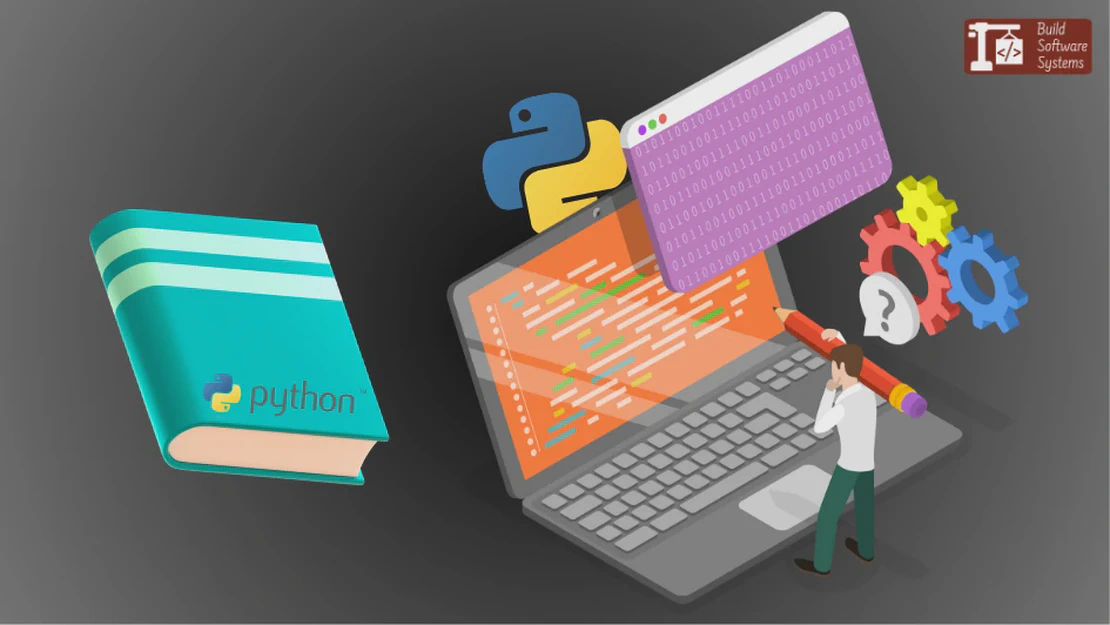
Python Learning Resources and Coding Conventions
- November 11, 2024
- 13 min read
- Python programming
Table of Contents
If you’re looking to learn the Python programming language and improve your coding skills, using the right resources and following solid coding conventions is essential.
This document offers a concise overview of key materials and tools to help you master Python programming while ensuring your code is consistent, maintainable, and readable.
Newsletter
Subscribe to our newsletter and stay updated.
​
Language Learning Resources
​
Syntax and Semantics
- Python for non-programmers: Beginner-friendly Python guide for users with no programming experience, featuring tutorials and foundational concepts.
- Getting started with Python: Official resource with installation steps and basic information to help new users quickly start coding in Python.
- Python Language Reference: The official Python language documentation covering syntax and semantics.
- Python Enhancement Proposals (PEPs): Official design documents outlining new features, language standards, and best practices for Python development.
​
Standard Library and Cross-Platform Resources
- Python Built-in and Standard Library Reference: A comprehensive guide to Python’s built-in and standard library, including modules for file handling, data manipulation, and networking.
You should check it before implementing common functionalities or considering third party library.
Newsletter
Subscribe to our newsletter and stay updated.
​
Coding Style and Best Practices
​
Best Practices
- PEP 20 - The Zen of Python: A set of aphorisms that capture the guiding principles of Python’s design, such as “Readability counts” and “There should be one—and preferably only one—obvious way to do it.”
- PEP 257 - Python Docstring Conventions: The standard for writing documentation within Python code. PEP 257 outlines conventions for docstrings, including how to document modules, classes, functions, and methods.
​
Coding Style Guides
Following consistent coding conventions is essential for readability and maintainability.
- PEP 8 - Style Guide for Python Code: The official Python style guide for writing readable and consistent Python code. PEP 8 is widely adopted across Python projects and defines conventions for naming, formatting, and structuring code.
- Google Python Style Guide: Google’s guide to writing clean and readable Python code, covering conventions, naming, imports, and more.
- Black’s Style Guide: Black is an opinionated code formatter for Python, and it follows specific conventions to ensure consistency.
​
Documentation Styles
Good documentation styles make it easy to understand code for future developers (and for your future self).
- Google Style Python Docstrings: Google’s docstring format offers structured sections for parameters, returns, and exceptions, making it highly readable and widely used. This is part of the “Google Python Style Guide”.
- reStructuredText (reST) Style: Used by Sphinx, this style supports complex documentation features like tables and cross-referencing, ideal for larger projects.
- Numpy Docstring Format: A structured format commonly used in scientific projects and libraries, this style includes sections for extended details and examples.
- Epydoc/Epytext: A docstring formats that make it easy to document classes, methods, and functions.
Tip
Whether you follow PEP 8 or use a formatter like Black, consistency is key. Stick with one style throughout your codebase to maintain readability and cohesiveness.
​
Error Handling Conventions
Effective error handling is crucial in Python for building resilient programs. Python’s official guidelines recommend specific structures for managing errors gracefully.
- Python’s Official Error Handling Tutorial: This tutorial explains the basics of Python’s error handling, covering
try
,except
,else
, andfinally
blocks, alongside best practices for writing robust error-handling code.
​
Naming Conventions
Clear and consistent naming conventions improve readability and help other developers understand your code more easily.
- PEP 8 Naming Conventions: PEP 8 provides naming conventions for different types of identifiers in Python, such as functions, variables, classes, and constants.
Newsletter
Subscribe to our newsletter and stay updated.
​
Resources for Specific Applications
​
Asynchronous Applications
Asynchronous programming is a core feature in Python for handling I/O-bound operations efficiently.
- Asyncio: Python’s built-in library for writing concurrent code using async/await, ideal for managing multiple tasks non-blockingly.
- uvloop: A high-performance event loop for asyncio, designed to boost asyncio’s speed by replacing the default event loop, especially for network-intensive applications.
- Trio: A modern Python async library designed to be simpler and easier to use than asyncio for high-level async concurrency.
- AnyIO: A library that provides a consistent API for asynchronous I/O, supporting asyncio, Trio, and other event loops.
​
Command-Line Interface (CLI) Development
Python makes it easy to build powerful and user-friendly command-line interfaces (CLI).
- argparse: The built-in Python library for handling command-line arguments, making it easy to write user-friendly CLI programs.
- Click: A package that provides a command-line interface toolkit for building complex CLI applications, with support for arguments, options, and subcommands.
- Typer: A library for building APIs and CLIs with type hints, based on FastAPI, designed to be easy to use while providing type safety.
​
Data Science and Machine Learning
Python is widely used in data science for tasks such as data manipulation, statistical analysis, and building machine learning models.
- NumPy: A package for scientific computing, offering support for large, multi-dimensional arrays and a wide array of mathematical functions.
- Pandas: A powerful library for data analysis and manipulation, designed to handle structured data with ease.
- scikit-learn: A tool for data mining and analysis, built on NumPy, SciPy, and matplotlib.
- TensorFlow: An open-source machine learning framework for building deep learning models and neural networks.
- PyTorch: A flexible and fast deep learning platform for research and deployment.
- Matplotlib: A comprehensive library for creating static, animated, and interactive visualizations, essential for data visualization in Python.
​
Game Development
Python can also be used for developing games, especially with libraries that simplify graphics, sound, and logic.
- Pygame: A set of Python modules designed for writing video games, providing functionality for graphics, sound, and event handling.
- Arcade: A modern Python framework for developing 2D games with an emphasis on simplicity and ease of use.
- Pyglet: A cross-platform windowing and multimedia library for Python, providing support for creating games and other visually rich applications.
​
GUI Development
If you’re looking to develop desktop applications with graphical interfaces, these Python libraries can help:
- Tkinter: The standard Python interface to the Tk GUI toolkit.
- PyQt: A set of Python bindings for the Qt application framework, useful for building cross-platform applications.
- Kivy: An open-source Python library for rapid development of applications with multi-touch support, often used for mobile and desktop development.
- wxPython: A popular Python library for creating native user interfaces, offering support for a wide range of platforms and tools.
- PyGObject: A Python binding for GTK, used for creating graphical user interfaces in Linux and other platforms with the GNOME toolkit.
If you are not satisfied with these, a more complete list can be found here.
​
Scientific Computing
For complex scientific computing, Python has a number of specialized libraries.
- SciPy: A library for scientific and technical computing, built on top of NumPy, and includes algorithms for optimization, integration, interpolation, and more.
​
Web Development
Python is a popular choice for building web applications, with frameworks that support both simple sites and complex, enterprise-level applications.
- Django: A high-level web framework that encourages rapid development and clean, pragmatic design. It’s known for its “batteries-included” philosophy and ease of use.
- Flask: A micro web framework that is flexible and lightweight, offering the essentials for web app development without imposing too many dependencies or structure.
- FastAPI: A modern web framework for building APIs with Python 3.7+ based on standard Python type hints. It’s designed to be fast, simple, and intuitive. It is based on JSON Schema and OpenAPI (API can be visualized using Swagger UI).
- aiohttp: A library for asynchronous HTTP requests and web servers, making it ideal for building high-performance, non-blocking web applications.
Newsletter
Subscribe to our newsletter and stay updated.
​
Tooling
​
Interpreters and Build Tools
​
Interpreters
- CPython: The default, widely-used implementation of Python, offering an interpreter with extensive standard libraries.
- PyPy: An alternative Python interpreter known for faster performance due to just-in-time (JIT) compilation. It’s compatible with CPython and ideal for applications that require high speed.
- MicroPython: A lightweight version of Python designed to run on microcontrollers and constrained environments, commonly used in embedded systems development.
​
Build Automation
Python offers robust tools for managing dependencies and automating build processes.
- Setuptools: A package used for building and distributing Python packages. It includes commands for packaging and installation.
- Poetry: A modern dependency manager and package builder for Python, Poetry simplifies the management of Python packages and dependencies with an intuitive configuration format.
- Makefile with Python: While Make is traditionally used for C/C++, it’s also helpful for automating Python tasks, such as setting up virtual environments and running tests.
​
Package Management and Virtual Environments
Efficient dependency management is essential for Python projects, especially when sharing code or deploying to different environments.
- pip: The default package installer for Python, used for installing libraries and managing dependencies.
- Conda: A powerful package manager for Python (and other languages) used in scientific and data science projects, supporting multiple environments and package isolation.
- Poetry: Also serves as a package manager, designed to simplify dependency management and project configuration.
- Pipenv: A packaging tool that aims to bring the best of all packaging worlds together, allowing users to set up a virtual environment, manage dependencies, and maintain a Pipfile.
- pip-compile-multi: A tool that extends pip-tools to support managing multiple requirements files and environments, allowing for better organization and control of dependencies.
- PBR (Python Build Reasonableness): A library designed to help Python projects comply with best practices for packaging, offering support for versioning, automatic changelog generation, and integrating with setuptools.
​
Documentation Generation Tools
- pydoc: A built-in Python tool for generating documentation from docstrings and viewing module documentation in the terminal.
- Sphinx: A documentation generator widely used in the Python community, especially for open-source libraries, creating output formats like HTML, LaTeX, and more.
- Epydoc: A Python-specific tool for generating API documentation from docstrings, ideal for documenting classes, functions, and modules in a structured, accessible format.
- pdoc: A simple and fast Python documentation generator that produces clean, user-friendly API documentation directly from docstrings.
- Pydoctor: A tool for generating API documentation for Python projects, designed for use with large codebases and provides support for documenting code automatically.
- MkDocs: A fast, simple static site generator that is ideal for creating project documentation using Markdown.
- Pandoc: A versatile document converter that supports numerous input and output formats, making it easy to convert between Markdown, reStructuredText, HTML, and others.
​
Linter and Formatting Tools
Using linters and formatters is key for consistent style and catching potential errors early.
- Pylint: A comprehensive Python linter that enforces PEP 8 standards, catches potential errors, and checks for code smells.
- Black: An automatic code formatter that reformats Python code to be consistent with an opinionated style guide, reducing code style debates.
- flake8: A tool that combines PEP 8 checks with error detection for better code consistency.
- isort: A tool to automatically sort Python imports according to style guides like PEP 8.
​
Debugging and Profiling Tools
Debugging and profiling tools help diagnose issues and optimize performance in Python.
- PDB (Python Debugger): The built-in Python debugger, allowing you to set breakpoints, inspect variables, and step through code interactively.
- PyCharm Debugger: A full-featured IDE with an integrated debugger, making it easier to set breakpoints, view variable states, and evaluate expressions.
- cProfile: A built-in profiling module that measures where time is spent in your code, helping optimize performance.
- memory_profiler: A package for tracking memory usage in Python code, useful for optimizing memory-intensive applications.
​
Unit Testing in Python
Testing is crucial to ensure your Python code works as expected.
- unittest: The built-in testing framework for Python, supporting test case organization, test discovery, and output reports.
- pytest: A powerful testing tool that makes it easy to write small tests, offers extensive plugins, and is compatible with
unittest
-style test cases. - doctest: A built-in module that allows testing by embedding expected output within docstrings.
doctest
helps ensure documentation stays accurate. - Asyncio Testing: Built-in support for testing asynchronous code, making it easier to test
asyncio
-based functions. - pytest-asyncio: A plugin for pytest that provides support for testing asynchronous code written with
asyncio
. It allows you to useasync
/await
syntax in your test functions. - pytest-timeout: A plugin for pytest that allows you to set a time limit for tests to run, ensuring they don’t hang indefinitely and providing a way to catch performance issues or infinite loops in your test code.
- Hypothesis: A property-based testing tool for Python that automatically generates test cases based on your code’s requirements.
Newsletter
Subscribe to our newsletter and stay updated.
​
Conclusion
By following these coding conventions and leveraging the resources listed, you’ll be well on your way to mastering Python programming.
Whether you’re just starting or looking to refine your skills, these tools and guidelines will help you write better, more maintainable, and efficient Python code.
Do you use relevant Python programming resources that haven’t been mentioned in this document?
Feel free to share them in the comments section or let us know!