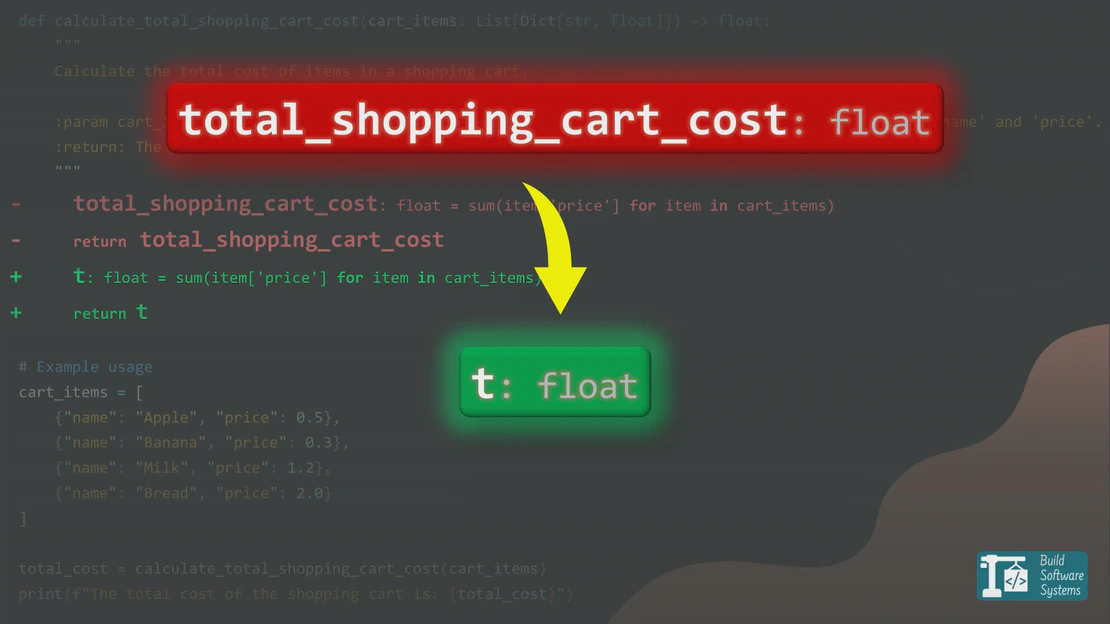
Descriptive Variable Names Are Not Always Good
- February 5, 2025
- 8 min read
- Programming concepts , Software quality
Table of Contents
When naming variables in a program, the usual advice is to use descriptive names.
The rationale is that descriptive names make code easier to understand, acting as self-documenting comments.
But is this always the case? Let’s explore when shorter, less descriptive names might actually improve readability.
​
The Case for Descriptive Variable Names
First, let’s look at two code snippets that are identical except for their variable names:
Snippet 1: Short Names
1def count_above_threshold(x: int, y: list[int]) -> int:
2 """
3 Count elements in the list greater than the threshold.
4 """
5 s: int = 0
6 for a in y:
7 if a > x:
8 s += 1
9 return s
Snippet 2: Long Descriptive Names
1def count_above_threshold(threshold: int, numbers: list[int]) -> int:
2 """
3 Count elements in the list greater than the threshold.
4 """
5 count: int = 0
6 for number in numbers:
7 if number > threshold:
8 count += 1
9 return count
At first glance, the second snippet is clearer because threshold
, numbers
, and count
describe their purpose explicitly.
In contrast, the first snippet uses cryptic names like x
, y
, and s
, forcing the reader to deduce their meaning.
But does this mean we should always use long, descriptive names? Not necessarily.
​
When Long Descriptive Names Backfire
While descriptive names help in most cases, there are situations where excessive verbosity can reduce clarity.
Consider a function that swaps elements across the main diagonal of a matrix:
1def diagonal_swap(x: list[list[float]]):
2 """
3 Swap elements across the main diagonal in the given matrix, in place.
4 """
5 n: int = len(x)
6 for i in range(n):
7 for j in range(i + 1, n):
8 x[i][j], x[j][i] = x[j][i], x[i][j]
Now, here’s the same function with longer, more descriptive variable names:
1def diagonal_swap(matrix: list[list[float]]):
2 """
3 Swap elements across the main diagonal in the given matrix, in place.
4 """
5 size: int = len(matrix)
6 for row_index in range(size):
7 for col_index in range(row_index + 1, size):
8 matrix[row_index][col_index], matrix[col_index][row_index] = \
9 matrix[col_index][row_index], matrix[row_index][col_index]
Which version is easier to read? Surprisingly, the first one, despite its short, non-descriptive variable names. Why?
While the longer names (row_index
, col_index
) do improve clarity, the unnecessary verbosity outweighs the benefits.
This scenario is more common in code that involves heavy mathematical operations, such as linear algebra or numerical computations.
Generic mathematical operations often don’t benefit from long descriptive variable names.
For example, using x
and y
in the following Python lambda function is completely fine:
1def product_list(list1: list[int], list2: list[int]):
2 return list(map(lambda x, y: x * y, list1, list2))
Expanding x
and y
into value1
and value2
doesn’t help readability:
1def product_list(list1: list[int], list2: list[int]):
2 return list(map(lambda value1, value2: value1 * value2, list1, list2))
So, how do you decide which short variable names to use? Does it even matter?
Newsletter
Subscribe to our newsletter and stay updated.
​
What Makes Short Variable Names Work?
The reason why the short-name version of diagonal_swap
is more readable isn’t just because the names are short and the code is less verbose.
There’s more to it: the short names are deliberately chosen.
Let’s rename some of the short names:
1def diagonal_swap(x: list[list[float]]):
2 """
3 Swap elements across the main diagonal in the given matrix, in place.
4 """
5 i: int = len(x)
6 for n in range(i):
7 for f in range(f + 1, i):
8 x[n][f], x[f][n] = x[f][n], x[n][f]
This version reads less naturally. Why?
I used i
to represent the number of rows, n
for the row index, and f
for the column index. This isn’t the usual way to represent these items.
Letters like i
, j
, and k
are commonly used for indices.
Similarly, in Python exception handling, e
is a common shorthand (idiomatic) name for exception:
1try:
2 # some code that may raise an exception
3except Exception as e:
4 print(e)
Renaming e
to something like exception_object
would only add unnecessary noise.
Hence, idioms matter when choosing short names. Following them makes code with short names more readable.
Tip
Avoid using an idiomatic variable name for something other than what it represents.
Let’s revisit the second snippet of the count_above_threshold
function with a slight tweak:
1def count_above_threshold(threshold: int, numbers: list[int]) -> int:
2 """
3 Count elements in the list greater than the threshold.
4 """
5 count: int = 0
6 for a in numbers:
7 if a > threshold:
8 count += 1
9 return count
Here, the loop variable a
is short, but the function parameters (threshold
, numbers
) and count
are descriptive. The code is still very readable.
The variable a
is just a loop variable, so its context is directly inferred from the corresponding variable numbers
. Hence, it doesn’t need a long, descriptive name.
Tip
When code is too verbose, you can selectively shorten some or all variables, starting with the most idiomatic ones or loop variables.
​
When Short Names Hurt Readability
There are cases where using short variables to reduce unnecessary verbosity can instead make the code harder to understand.
Consider this example:
1...
2 # Update total distance and fuel used
3 total_distance += distance
4 total_fuel_used += fuel_used
5 n += 1
6
7# Calculate fuel efficiency for each vehicle
8for vehicle_id, metrics in trip_metrics.items():
9...
What is n
? The context isn’t clear. Renaming it makes the purpose obvious:
1...
2 # Update total distance and fuel used
3 total_distance += distance
4 total_fuel_used += fuel_used
5 trip_counts += 1
6
7# Calculate fuel efficiency for each vehicle
8for vehicle_id, metrics in trip_metrics.items():
9...
The problem here is that the purpose of the variable n
isn’t embedded in its name. As a result, the reader must scroll back to understand its meaning, disrupting the flow of reading.
To mitigate this problem, some developers advocate for the scope length rule:
In scenarios where a variable’s scope is limited to a small portion of your code and its usage is immediately evident, concise names are acceptable.
This rule suggests that short, non-descriptive names should only be used when the scope is small, and the reader can quickly and easily see what the variable is used for without needing to look elsewhere in the code or add additional explanation.
In this case, even if long descriptive names are unnecessarily verbose, the clarity they provide outweighs the lack of contextual information from short variables.
Tip
If a variable’s meaning isn’t clear without scrolling, it needs a better name.
To reduce verbosity, consider refactoring the code (e.g., adding intermediate variables).
There’s another crucial consideration that can sometimes override the scope length rule or the need to improve the clarity of a single variable name: consistency.
​
Consider Consistency
One important consideration when naming variables is consistency.
If your team has agreed to use i
and j
for indices, deviating from this convention (e.g., using row_index
and col_index
) might cause confusion, and vice versa.
For example, in the Python ecosystem, libraries like NumPy and Pandas use short names like np
and df
consistently.
These names are widely understood and accepted because they follow community conventions. Sticking to such conventions ensures that your code is readable and maintainable by others.
Another consideration is that variable naming conventions can vary across programming languages.
For instance, in functional programming languages like Haskell, single-letter names like x
and xs
(for a list) are common and accepted.
In contrast, in Java, more descriptive names are often preferred unless the context is clear.
Tip
When consistency makes your code less readable, refactor. For example, if you conventionally use i
and j
for loop indices but have a very large loop, consider breaking it into smaller functions or using more descriptive names to improve clarity.
Newsletter
Subscribe to our newsletter and stay updated.
​
Conclusion
Using long, descriptive names isn’t always the best approach. Sometimes, short names make the code cleaner.
Follow these guidelines:
- Prioritize consistency.
- Use short (mathematical) names for generic math operations.
- Use long descriptive variable names when the code won’t become overly verbose.
- Use short names for loops, indices, and well-known conventions (
i
,j
,k
,e
), where descriptive names would make the code unnecessarily verbose. - When using short names, use idiomatic names appropriately.
- Avoid short names if a name lacks context and forces readers to scroll.
This applies mostly to variables. Other elements like classes, functions, and enums should always have clear, descriptive names.
This article was inspired by Tom Kwong’s blog on naming things properly. It explores why longer names sometimes make code harder to read and when short names work better.
Do you know other cases where short names improve readability? Share your thoughts in the comments!