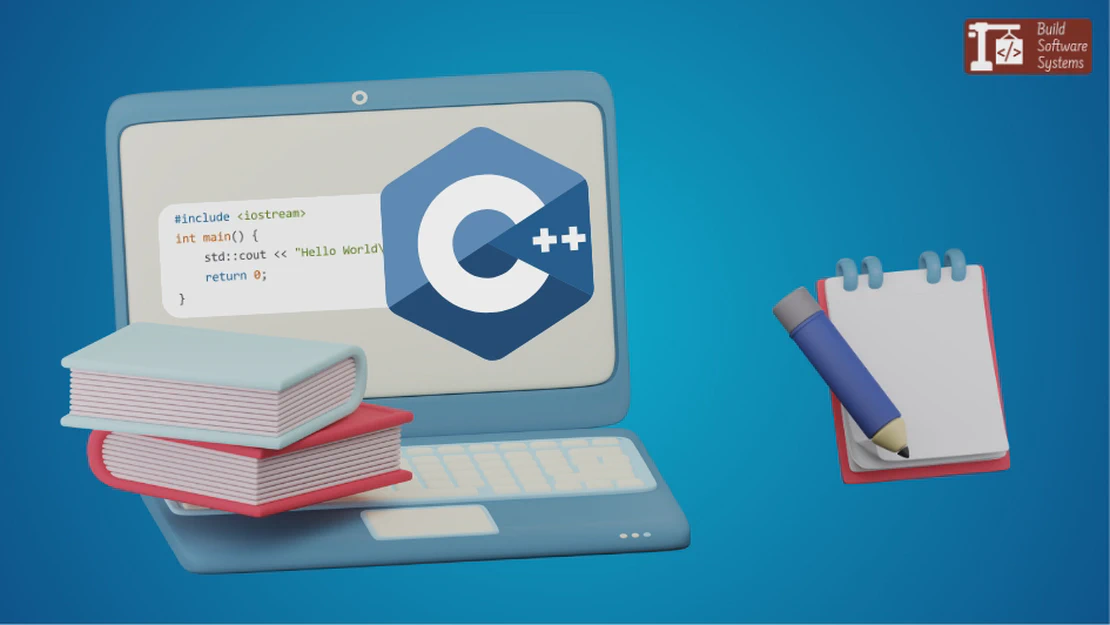
C++ Learning Resources and Coding Conventions
- October 20, 2024
- 8 min read
- C++ programming
Table of Contents
If you’re looking to learn the C++ programming language and improve your coding skills, using the right resources and following solid coding conventions is essential.
This document offers a concise overview of key standards, tools, and materials to help you master C++ programming while ensuring your code is consistent, maintainable, and readable.
Newsletter
Subscribe to our newsletter and stay updated.
​
C++ Language Learning Resources
​
Syntax, Semantics, and Language Standards
- ISO C++ Standard: The official standardization site for C++, including drafts (e.g.: year 2020 PDF draft) and documentation for the latest C++ standards.
- C++ Language Reference: A community-maintained resource providing detailed information on C++ syntax, semantics, and the standard library.
- GNU C++ Reference Manual: Documentation for the GNU implementation of C++.
- Microsoft C++ Language Reference: Official documentation for Microsoft’s implementation of C++, offering comprehensive information on syntax, keywords, and language constructs.
​
Standard Library and Cross-Platform Resources
- C++ Standard Library Reference: A comprehensive guide to the C++ standard library, covering containers, algorithms, and utilities.
- Windows C++ Runtime Library (CRT) Reference: Microsoft’s C++ runtime documentation, providing essential functions for input/output, memory management, and error handling.
- POSIX Standard for C++: Crucial for writing cross-platform C++ programs, particularly when targeting UNIX-like systems.
- Boost C++ Libraries: A set of peer-reviewed, portable C++ libraries that extend the functionality of the C++ standard library. Boost provides a wealth of useful tools like smart pointers, regular expressions, and threading.
​
System Programming and System Calls
Understanding system calls and low-level programming is essential for efficient C++ code, particularly for system-level operations.
- Linux System Calls: Detailed documentation of Linux system calls.
- Linux Man Pages: A comprehensive resource for Linux C++ APIs and related utilities.
- Windows System Calls: Documentation for Windows system calls, helpful for system-level C++ programming on Windows platforms.
Newsletter
Subscribe to our newsletter and stay updated.
​
Coding Style and Best Practices
​
Best Practices
- SEI CERT C++ Coding Standard: A coding standard that promotes secure coding practices in C++, helping you avoid common vulnerabilities like buffer overflows, race conditions, and memory leaks.
​
Coding Style Guides
Consistency is crucial for making C++ code more readable and maintainable. Here are key resources for coding style, naming conventions, and documentation.
- Google C++ Style Guide: A widely respected guide to C++ best practices, covering everything from naming conventions to code structure and comments.
- LLVM Coding Standards: A comprehensive coding standard for the LLVM project that emphasizes clarity, consistency, and performance.
- Boost C++ Libraries Coding Guidelines: A set of guidelines used for developing the Boost libraries, encouraging clean, maintainable, and efficient C++ code.
Tip
Whether you follow a formal standard like Google’s or LLVM’s guidelines, or develop your own, consistency is key. Stick with one style throughout the codebase to maintain readability and cohesiveness.
Newsletter
Subscribe to our newsletter and stay updated.
​
Tooling
​
Compilers and Build Tools
​
Compilers
- GNU Compiler Collection (GCC): Available on Linux, macOS, and Windows (via MinGW), GCC is one of the most popular open-source C++ compilers.
- LLVM Clang: Clang is a fast, modern C++ compiler supporting cross-platform development. It is particularly known for producing clear, informative error messages.
- Microsoft Visual Studio: An integrated development environment (IDE) for Windows, providing a powerful C++ compiler, excellent debugging tools, and performance analysis.
​
Build Automation
- CMake: A cross-platform build tool that simplifies the generation of build files for C++ projects, supporting different platforms, including Linux, macOS, and Windows.
- Autotools: A collection of tools that help automate the build process for portable C++ code, especially on Unix-like systems.
- Ninja: A small build system that focuses on speed and is often used as a backend build tool in combination with CMake.
- GNU Make: A widely-used build automation tool, it reads a makefile to understand dependencies between files and automates the compilation process.
​
Package Management
Managing libraries and dependencies is a key part of modern C++ development. These package managers make it easier to include external libraries.
- Conan: A popular, decentralized package manager for C/C++ that supports all platforms and build systems. Conan is ideal for managing and reusing dependencies across various projects.
- vcpkg: A package manager developed by Microsoft to simplify library management in C/C++ projects, with extensive support for cross-platform development.
- Hunter: A cross-platform CMake package manager that allows for seamless integration of dependencies.
​
Documentation Generation Tools
- Doxygen: The most widely used documentation generator for C++ code, supporting various output formats like HTML and LaTeX.
- Sphinx: A tool originally for Python, but with plugins that support generating documentation for C++ projects.
- Pandoc: A universal document converter that supports various formats such as Markdown, LaTeX, and HTML, making it a versatile tool for generating documentation.
- MkDocs: A fast and simple static site generator for creating project documentation, written in Markdown.
​
Linter and Formatting Tools
Maintaining a consistent code style and catching errors early is crucial in C++ development.
- Clang-Tidy: A C++ linter that flags common mistakes, suggests fixes, and ensures your code follows best practices.
- Clang-Format: A tool for automatically formatting your C++ code according to custom or predefined style rules.
- Cppcheck: A static analysis tool that helps identify bugs and performance bottlenecks in C++ programs.
- Uncrustify: A customizable formatting tool that ensures consistent code style across large C++ codebases.
​
Debugging and Profiling Tools
Effective debugging and profiling tools help diagnose issues and optimize your C++ applications.
- GDB (GNU Debugger): A powerful, open-source debugger that helps you inspect variables, set breakpoints, and step through C++ code.
- Valgrind: A suite of tools for memory debugging, memory leak detection, and profiling.
- Visual Studio Debugger: A highly capable debugger for C++ applications on Windows, with support for breakpoints, call stacks, and variable inspection.
​
Unit Testing in C++
Unit testing helps verify that your C++ code behaves as expected.
- Google Test (gtest): A powerful, easy-to-use C++ testing framework that supports mocking and integration with CI pipelines.
- Catch2: A lightweight and modern unit testing framework for C++, emphasizing simplicity and ease of use.
- Boost.Test: Part of the Boost library, providing a comprehensive framework for writing unit tests in C++.
Newsletter
Subscribe to our newsletter and stay updated.
​
Conclusion
By following these coding conventions and using the listed resources, you’ll be well on your way to mastering C++ programming. Whether you’re just starting or looking to refine your skills, these tools and guidelines will help you write cleaner, more efficient, and maintainable C++ code.
Do you use relevant C++ programming resources that haven’t been mentioned in this document?
Feel free to share them in the comments section or let us know.